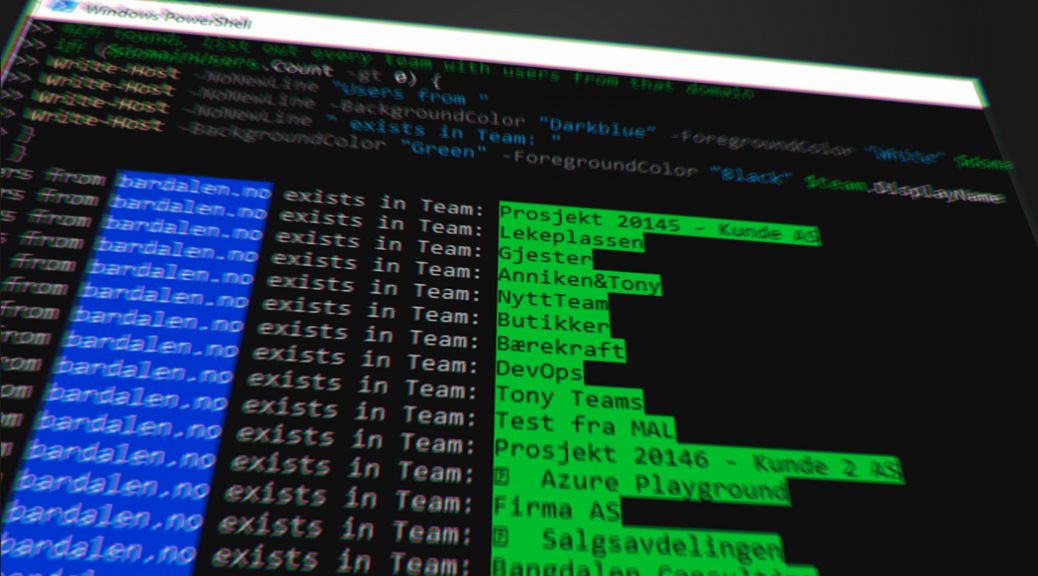
List Teams with users from specific domains
You have probably been in this situation where you need to migrate a sub-set of users from one tenant to another. This typically happens when a company for a reason splits out and only some users will be migrated. How are you going to get a list of the Teams they are a member of so you can begin migrate the correct data?
Fear not! I’ve recently been in this situation where the users already had a specific domain as their UPN and came up with a script that list only Teams they are a member of. Let me just paste it in here in all it’s glory:
#Set the domain you are searching for
$domain = "domain.org"
#Import module for Teams
Import-Module -Name MicrosoftTeams
#Connect to Microsoft Teams
Connect-MicrosoftTeams
#List out every team with every member
$AllTeams = Get-Team
#Loop through every Team and sort out users
Foreach ($team in $AllTeams)
{
#List out every user in that team
$teamUsers = Get-TeamUser -groupid $team.groupid
#Finds users with the specified domain
$domainUsers = $teamUsers | Where-Object { $_.User.EndsWith($domain) }
#If found, list out every team with users from that domain
if ($domainUsers.Count -gt 0) {
Write-Host -NoNewLine "Users from "
Write-Host -NoNewLine -BackgroundColor "Darkblue" -ForegroundColor "White" $domain
Write-Host -NoNewLine " exists in Team: "
Write-Host -BackgroundColor "Green" -ForegroundColor "Black" $team.DisplayName
}
}
#Disconnect
Disconnect-MicrosoftTeams
Link to my GitHub: https://github.com/Bangdalen/PS-MS365-Tools/blob/master/Get-TeamsWithSpescificUsersWithSpecificDomain.ps1
First we define our domain with the variable $domain.
$domain = "domain.org"
Then we import the module for Microsoft Teams
Import-Module -Name MicrosoftTeams
If you don’t have this module, just install it with:
Install-Module -Name MicrosoftTeams
Then we can connect to Teams and log in with an admin
Connect-MicrosoftTeams
After it connects it will list out every Team and their IDs to a variable called $AllTeams
$AllTeams = Get-Team
After that it loops through every Team in that variable and lists out every user to a new variable called $teamUsers
$teamUsers = Get-TeamUser -groupid $team.groupid
Alternatively you can add what kind of Role you are after, like this
$teamUsers = Get-TeamUser -groupid $team.groupid -Role "Owner"
Then the magic starts. The script filters out members based on what domain the UPN contains of into another variable called $domainUsers
$domainUsers = $teamUsers | Where-Object { $_.User.EndsWith($domain) }
The final task is to see if the variable $domainUsers are larger than 0, which means that Team are having members with that domain in their UPN. If the value is 1 or higher, it will inform that users with this domain exists in this Team
if ($domainUsers.Count -gt 0) {
...
}
That’s it really. Maybe I will expand this script to check for multiple domains, differentiate between different roles and other types of filtering in the future.