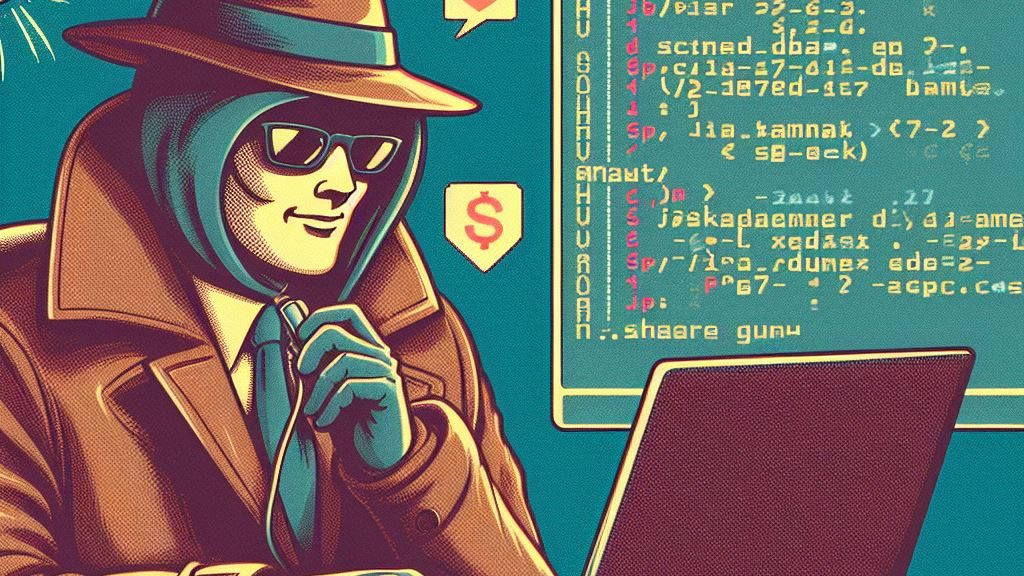
Create teams and channels in bulk
Thanks to the magic of PowerShell and JSON, we can add teams and channels in bulk! Had to do a project lately with a lot of teams and channels that needed to be created in a way that minimizes errors and potential screw-ups. Since I’m all for automating these kinds of tasks, I created this project that I want to share with you!
You do need some prerequisites:
- Some knowledge of both PowerShell and JSON
- The Teams-module installed on your computer
- Rights to a tenant to create teams
- Some teams and channels that needs to be created!
Create the JSON-file
First, you need a source for what structure you want to generate. This is my template JSON-file:
[
{
"TeamName": "Team1",
"Channels": [
{
"ChannelName": "Channel1",
"MembershipType": "Private"
},
{
"ChannelName": "Channel2",
"MembershipType": "Standard"
}
]
},
{
"TeamName": "Team2",
"Channels": [
{
"ChannelName": "Channel3",
"MembershipType": "Private"
},
{
"ChannelName": "Channel4",
"MembershipType": "Standard"
}
]
}
]
Let me explain the structure. First we define what teams we need to be created. They are in the first level in the JSON-file. Under each team there is an array with all the channels belonging to that team. Note: this is in addition to the General-channel all teams must have.
Each channel must have a name, and a defined membership type. The types I use here are “Standard” and “Private”. You can also create “Shared”, but I will not cover it at this point.
The PowerShell script
First, we begin with the besics of settings things up. We define the root folder from where you run the script and where everything resides. I know there are ways to do this in a more slick way. Maybe in a future version this will be more dynamic?
We point to the JSON file, read out the JSON file and converts the content from JSON to something PowerShell can read into a variable we later read out from.
Then we get some timestamps defined and sets up a log file to write to. Yeah, we log every bit to a file so you later can document what has happened!
# Read the JSON file
$rootFolder = "C:\data\"
$jsonFile = "Teams-and-Channels.json"
$jsonData = Get-Content $jsonFile | ConvertFrom-Json
$timestamp = (Get-Date).toString("yyyyMMddHHmm")
$logFileName = "Log_" + $timestamp + ".txt"
$logFileAndPath = $rootFolder + $logFileName
New-Item -Path $rootFolder -Name $logFileName -type file
The next part tries to connect to Teams. And of course logs the results, even if it fails. Remember to use a user that has access to create Teams!
try {
Connect-MicrosoftTeams
$messageTimestamp = (Get-Date).toString("yyyyMMdd-HH:mm:ss")
$message = "Successfully connected to Microsoft Teams!"
Write-Host $messageTimestamp - $message
} catch {
$messageTimestamp = (Get-Date).toString("yyyyMMdd-HH:mm:ss")
$message = "Could not connect to Microsoft Teams!"
Write-Host $messageTimestamp - $message
}
Add-Content -Path $logFileAndPath -Value "$messageTimestamp - $message"
The script goes through every bit in the now converted JSON data in the foreach-loop and creates a team. For every team you have defined, it reads the name and looks up if there’s a team with that name already. If that name is taken, it will throw an error about that. But! It will create channels in that team later on in the script!
When a team is created it will be created as a private team with a default description of “Team created via PowerShell”. Something we can customize in a later version?
After each team is created we wait 20 seconds. This is for the team to be properly created in the cloud before we can add channels.
# Iterate through each team in the JSON file
foreach ($teamData in $jsonData) {
$teamName = $teamData.TeamName
# Create Team if not already exists
if (-not (Get-Team -DisplayName $teamName)) {
New-Team -DisplayName $teamName -Description "Team created via PowerShell"
$messageTimestamp = (Get-Date).toString("yyyyMMdd-HH:mm:ss")
$message = "Created Team: " + $teamName
Write-Host $messageTimestamp - $message
Add-Content -Path $logFileAndPath -Value "$messageTimestamp - $message"
}
#Wait for the team to be created before adding channels
Write-Host "Waiting 20 seconds for the team to be properly created..."
Start-Sleep -Seconds 20
Write-Host "The wait is over. Continuing with the channels"
Another foreach loop is used to loop through the channels defined in the JSON file. If a channels does exist it will skip it and gop to the next. The result is of course logged to file.
foreach ($channelData in $teamData.Channels) {
$channelName = $channelData.ChannelName
$membershipType = $channelData.MembershipType
# Check if channel already exists and creates it
try {
New-TeamChannel -GroupId $teamId -DisplayName $channelName -Description "Channel created via PowerShell" -MembershipType $membershipType
$messageTimestamp = (Get-Date).toString("yyyyMMdd-HH:mm:ss")
$message = "Created channel: " + $channelName
Write-Host $messageTimestamp - $message
} catch {
$messageTimestamp = (Get-Date).toString("yyyyMMdd-HH:mm:ss")
$message = "Could not create channel. Already exists?: " + $channelName
Write-Host $messageTimestamp - $message
}
Add-Content -Path $logFileAndPath -Value "$messageTimestamp - $message"
}
}
Lastly we disconnect from Teams:
# Disconnect from Microsoft Teams
Disconnect-MicrosoftTeams
$messageTimestamp = (Get-Date).toString("yyyyMMdd-HH:mm:ss")
$message = "Disconnected from Microsoft Teams!"
Write-Host $messageTimestamp - $message
Add-Content -Path $logFileAndPath -Value "$messageTimestamp - $message"
The script is tested in controlled environments. Some parts are quite rudimentary but does the job. Besides, I’m not fluent in PowerShell so this is a great way for me to practise!
Links to my GitHub where this project is located:
https://github.com/Bangdalen/PS-MS365-Tools/tree/master/Generate-TeamsAndChannels
Feel free to either contribute or point out my mistakes 😀